Implementing the Checkout Page
The process required to implement the checkout page
In this document, we will outline how partners can integrate the checkout page for their merchants.
Below is a simple diagram on the checkout page process.
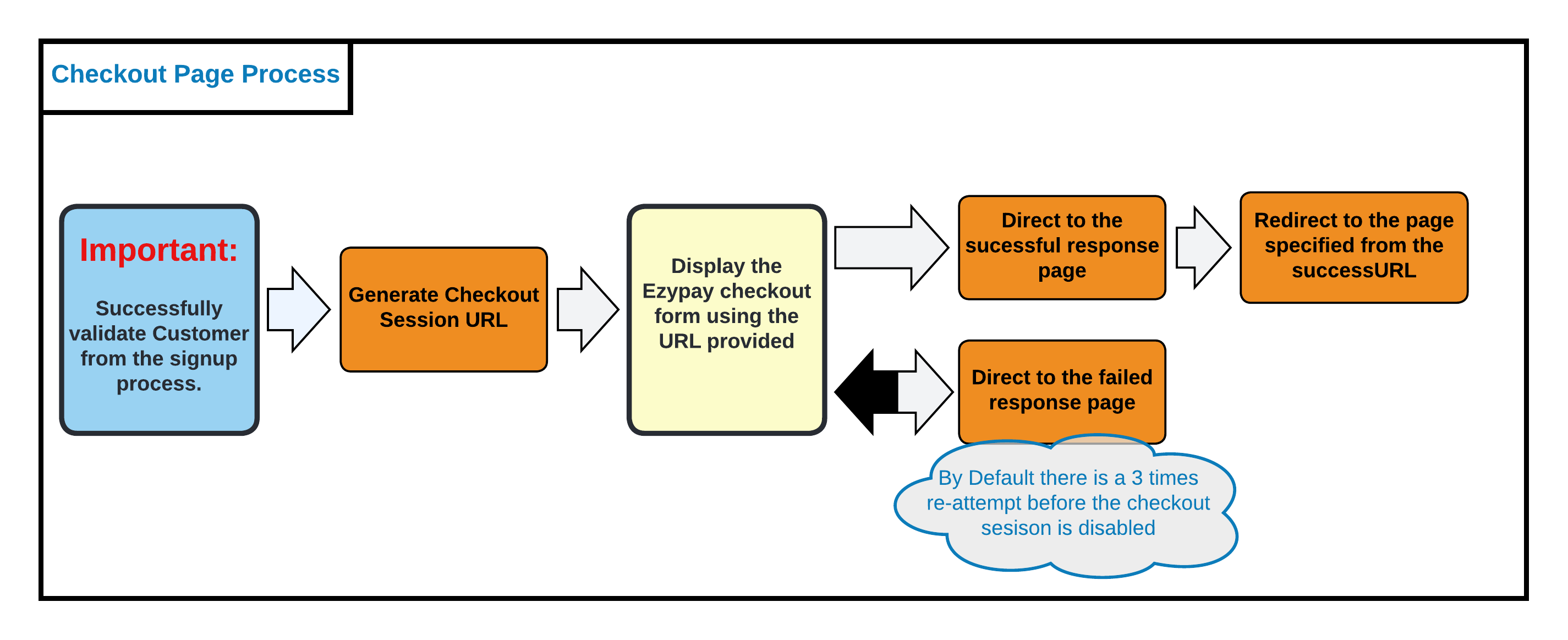
Checkout page API process
The checkout page allows merchants to collect once off payments via an URL link, there is no need to create a customer id and payment method token in advance.
The checkout URL that gets created will allow the customer to enter their own details and payment method before a payment can be made.
The required API parameters are listed in our API reference section,
"Create A Checkout session"
https://developer.ezypay.com/reference#create-a-checkout-session
NOTE:
- The checkout page only accepts credit card payments.
- The checkout URL created can be sent in an email or embedded into a website.
- Ensure you have a success URL that can accept parameters in the URL.
- Currently the concept of the checkout page developed by Ezypay is to have one unique invoice per checkout session id.
- The same checkout id should not be reused for a future invoice.
Creating a CheckoutURL
The most basic setup for creating a checkoutURL is passing in the Amount and Description, but it is important that ensure that you you have the CancelURL and SuccessURL parameter defined as well.
The SuccessURL will include details of the successful transaction. Having an active listening server means you will be able to know if the payment was processed successful in an immediate manner. ( https://developer.ezypay.com/docs/implementing-the-checkout-page#b-retrieving-checkout-id-from-invoice-status-webhook-events)
Ensuring you subscribe to the appropriate webhook (Invoice_Created, Invoice_Paid and Invoice_Past_Due) will also give you up to date status of the payment.
Compulsory Parameters
The following data are compulsory parameters for https://api-global.ezypay.com/v2/billing/checkout
Parameters | Request body |
---|---|
Amount | "amount": { "currency": "AUD", "value": 3.00 } |
Description | "description": "Orange Juice 250ml" |
CustomerId | By providing the Ezypay Customer Id, the checkout page will be preconfigured with the customer Firstname, Lastname, Email and Phone details. The fields will be disabled and cannot be changed. Its important to always have the customer validated before the checkout session url is provided to the customer. This ensure that the checkout payment collection was targeted to a specific/identified customer. { "customerId": "{{Ezypay Customer UUID}" } |
successUrl | The URL provided here will ensure the successful payment will be redirected to a specified page decided by the merchant. It is important to add this if you want to track these payments. Details of the transaction will be returned (Invoice Id, Customer Id, Payment method token and checkout Id). { "successUrl": "www.ezypaySuccessURL.com" } |
Optional Parameters
The following items are optional parameters.
These options allow you to:
-
- Link existing customer details to the once-off payment
or
- Link existing customer details to the once-off payment
-
- Provide an option to use an existing payment method
Optional items | JSON request |
---|---|
accountingCode | Can be used as an identifier on the merchant settlement report. The accountingCode can also be used to differentiate different revenue sharing arrangements. { "accountingCode": "test11234" } |
cancelUrl | If a customer cancels from the checkoutURL the page will redirect the customer to this URL upon cancelling of the checkout page. { "cancelUrl": "www.ezypay.com" } |
paymentMethodToken | Will ensure that the customer will only be able to pay using the payment method token associated with this payment method token. |
How to create a checkout page for new customers
[POST] https://api-sandbox.ezypay.com/v2/billing/checkout
API Endpoint Documentation: https://developer.ezypay.com/reference#create-a-checkout-session
{
"amount": {
"currency": "AUD",
"value": 3.00
},
"description": "Orange Juice 250ml",
"successUrl": "www.ezypaySuccessURL.com"
}
{
"checkoutUrl": "https://checkout-sandbox.ezypay.com/558450ff",
"id": "908fd0b1-86ff-4123-961c-4099210102d8"
}
How to create a checkout page with an existing customer id & payment method
[POST] https://api-sandbox.ezypay.com/v2/billing/checkout
{
"amount": {
"currency": "AUD",
"value": 100.00
},
"description": "Test1234567",
"customerId": "{{Existing Customer Id}}",
"paymentMethodToken": "{{Existing Payment Method Token}}",
"successUrl": "https://www.ezypay.com/"
}
{
"checkoutUrl": "https://checkout-sandbox.ezypay.com/dfc3e307",
"id": "e7e679e6-5be2-419f-a927-d86db6d702ef"
}
Identifying checkout id from the "successURL" & webhook events
A. Retrieving checkout Id from the success URL
https://www.ezypaysuccessurl.com/? **invoiceId**={{Invoice UUID}}&**customerId**={{Customer UUID}}&**paymentMethodToken**={{Payment Method UUID}}&**checkoutId**={{checkoutId}}

Example of the SuccessfulURL Redirecting
B. Retrieving checkout id from invoice status webhook events
Items to look out for when retrieving webhook events from checkout session invoices.
-
- checkoutId
-
- channel
{
"requestId": "63fa1370-de7f-4adb-bcb2-755446b99c9f",
"merchantId": "1aefbba9-263a-484f-8ba1-1b074b8c1493",
"eventType": "INVOICE_CREATED",
"createdOn": "2020-05-11T02:43:17.602",
"data": {
"id": "070d96b4-fc11-4274-9c8b-e9ba59fd3917",
"documentNumber": "IN0000000000001508",
"date": "2020-05-11",
"dueDate": "2020-05-11",
"scheduledPaymentDate": null,
"status": "PROCESSING",
"memo": null,
"items": [
....................................................
],
"amount": {
"currency": "AUD",
"value": 5.28,
"type": null
},
"amountWithoutDiscount": {
"currency": "AUD",
"value": 5.28,
"type": null
},
"totalDiscounted": {
"currency": "AUD",
"value": 0,
"type": null
},
"totalRefunded": {
"currency": "AUD",
"value": 0,
"type": null
},
"totalTax": {
"currency": "AUD",
"value": 0.48,
"type": null
},
"customerId": "42fd7bda-f867-49de-b4d5-26794012dc2a",
"subscriptionId": null,
"checkoutId": "908fd0b1-86ff-4123-961c-4099210102d8",
"subscriptionName": null,
"paymentMethodToken": "49c4b777-f04b-4bc4-b901-162492981df0",
"autoPayment": true,
"processingModel": "CARD_ON_FILE",
"createdOn": "2020-05-11T02:43:17.369",
"payNowUrl": null,
"channel": "online_checkout"
}
}
{
"requestId": "b1edda7e-7b87-4789-adbe-c9e59a5e64a0",
"merchantId": "1aefbba9-263a-484f-8ba1-1b074b8c1493",
"eventType": "INVOICE_PAID",
"createdOn": "2020-05-11T02:43:19.554",
"data": {
"id": "070d96b4-fc11-4274-9c8b-e9ba59fd3917",
"documentNumber": "IN0000000000001508",
"date": "2020-05-11",
"dueDate": "2020-05-11",
"scheduledPaymentDate": null,
"status": "PAID",
"memo": null,
"items": [
............
],
"amount": {
"currency": "AUD",
"value": 5.28,
"type": null
},
"amountWithoutDiscount": {
"currency": "AUD",
"value": 5.28,
"type": null
},
"totalDiscounted": {
"currency": "AUD",
"value": 0,
"type": null
},
"totalRefunded": {
"currency": "AUD",
"value": 0,
"type": null
},
"totalTax": {
"currency": "AUD",
"value": 0.48,
"type": null
},
"customerId": "42fd7bda-f867-49de-b4d5-26794012dc2a",
"subscriptionId": null,
"checkoutId": "908fd0b1-86ff-4123-961c-4099210102d8",
"subscriptionName": null,
"paymentMethodToken": "49c4b777-f04b-4bc4-b901-162492981df0",
"autoPayment": true,
"processingModel": "CARD_ON_FILE",
"createdOn": "2020-05-11T02:43:17.369",
"payNowUrl": null,
"channel": "online_checkout"
}
}
{
"requestId": "e7e9f4af-54a7-4e94-9bf8-1fa012903c29",
"merchantId": "1aefbba9-263a-484f-8ba1-1b074b8c1493",
"eventType": "INVOICE_PAST_DUE",
"createdOn": "2020-05-11T02:19:35.632",
"data": {
"id": "e4ab71ef-ba49-4f81-9dd4-ae27827ad893",
"documentNumber": "IN0000000000001506",
"date": "2020-05-11",
"dueDate": "2020-05-11",
"scheduledPaymentDate": null,
"status": "PAST_DUE",
"memo": null,
"items": [
......................................................
],
"amount": {
"currency": "AUD",
"value": 5.24,
"type": null
},
"amountWithoutDiscount": {
"currency": "AUD",
"value": 5.24,
"type": null
},
"totalDiscounted": {
"currency": "AUD",
"value": 0,
"type": null
},
"totalRefunded": {
"currency": "AUD",
"value": 0,
"type": null
},
"totalTax": {
"currency": "AUD",
"value": 0.47,
"type": null
},
"customerId": "3dcddf73-2450-439a-ae77-f4a819881242",
"subscriptionId": null,
"checkoutId": "908fd0b1-86ff-4123-961c-4099210102d8",
"subscriptionName": null,
"paymentMethodToken": "72c1ef48-dc05-4607-b8b6-dfaddce2a253",
"autoPayment": false,
"processingModel": null,
"createdOn": "2020-05-11T02:19:34.254",
"failedPaymentReason": {
"code": "invalid_payment_method",
"description": "Payment method details are invalid. Please use a different payment method."
},
"paymentProviderResponse": {
"code": "Refused",
"description": "Invalid card number"
},
"payNowUrl": null,
"channel": "online_checkout",
"manualRetryPossible": false,
"paymentMethodInvalid": true
}
}
Comparison between a normal on-demand invoice created VS checkout page invoice
Behaviour of the invoices:
Checkout page invoice | On-demand invoice |
---|---|
No auto re-bill through Ezypay and no failed payment notification/pay now link will take place even if the merchant has opted for this feature to be enabled in Ezypay. | Follows the failed payment settings setup for a merchant (auto-rebill through Ezypay and failed payment notification/paynow link). |
Transaction fees charges and the removal of any failed payment fees on that invoice can be different from normal on-demand settings. | |
"Channel" in the response body will state "online_checkout". | "Channel" in the response body will state "api". |
The "CheckoutId" will be returned. | No "CheckoutId" will be returned. |
Important
It's very important for integrators to be able to differentiate an on-demand invoice and an invoice generated from the checkout page to be able to handle these conditions differently. The best method to do that is by using the "Channel" and the "CheckoutId" details return from the API response details.
Updated 11 months ago